What is NLog?
NLog is a flexible and free logging platform for various .NET platforms, including .NET standard. NLog is easy to apply and it includes several targets (database, file, event viewer).
Which platform support it?
.NET Framework 3.5, 4, 4.5, 4.6 & 4.7
.NET Framework 4 client profile
Xamarin Android
Xamarin iOS
Windows Phone 8
Silver light 4 and 5
Mono 4
ASP.NET 4 (NLog.Web package)
ASP.NET Core (NLog.Web.AspNetCore package)
.NET Core (NLog.Extensions.Logging package)
.NET Standard 1.x - NLog 4.5
.NET Standard 2.x - NLog 4.5
UWP - NLog 4.5
There are several log levels.
Fatal : Something terrible occurred; the application is going down
Error : Something fizzled; the application might possibly proceed
Warn : Something surprising; the application will proceed
Info : Normal conduct like mail sent, client refreshed profile and so on.
Debug : For troubleshooting; the executed question, the client confirmed, session terminated
Trace : For follow troubleshooting; start technique X, end strategy X
Where we can log?
Console
Database
Email
Event Viewer Log
Files
Network
Implementation of NLog
Step 1 : Open Visual Studio. Select File > New > Project.
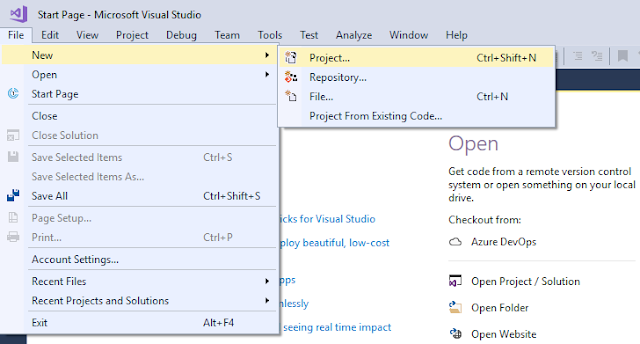
Step 2 : Select Web> ASP.NET Web Application > Give your project name "NLogTestApp", and then click OK.
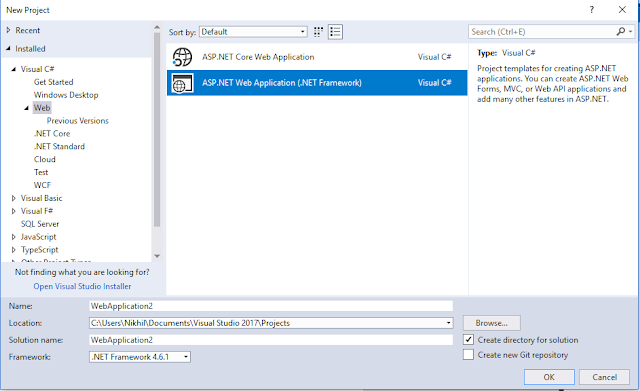
Step 3 : Select Web API and click OK.
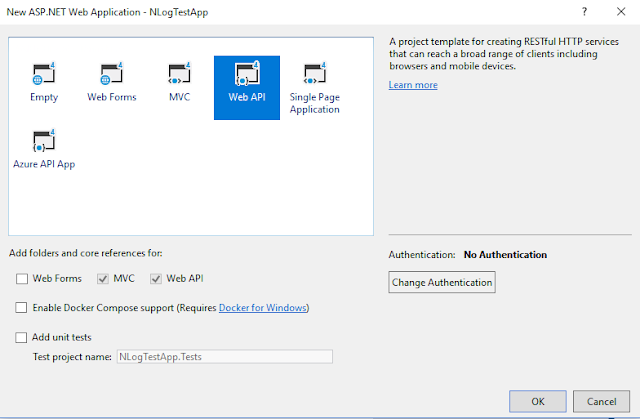
Step 4 : Now, we add NLog in our project. Right-click on your project solution, click on "Manage NugGet Packages". Click on the "Browse" link button and in the search box, type nlog. It will show you NLogDll as shown below. Just install this dll.
After that, when you click "Install", you will get the following window, i.e., Preview Changes.
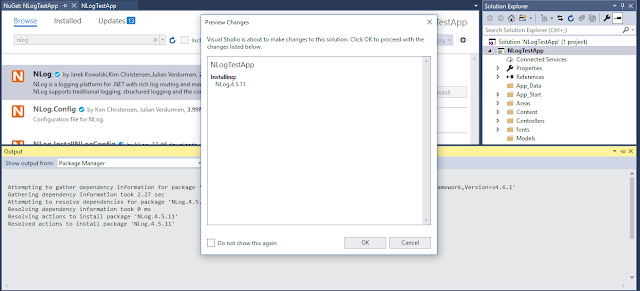
In this popup window, click the OK button. After it is successfully installed, you will get the Nlog dll in your project reference.
Step 5 : Now, add the following code to your config file.
<configSections>
<section name="nlog" type="NLog.Config.ConfigSectionHandler, NLog" />
</configSections>
<nlog xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<targets>
<target name="logfile" xsi:type="File" fileName="${basedir}/MyLogs/${date:format=yyyy-MM-dd}-api.log" />
<target name="eventlog" xsi:type="EventLog" layout="${message}" log="Application" source=" My Custom Api Services" />
<target name="database" type="Database" connectionString="Data Source=SRKSUR5085LT\SQLEXPRESS;initial catalog=NLog;user id=sa;password=OPTICAL;MultipleActiveResultSets=True;">
<commandText> insert into ExceptionLog ([TimeStamp],[Level],Logger, [Message], UserId, Exception, StackTrace) values (@TimeStamp, @Level, @Logger, @Message, case when len(@UserID) = 0 then null else @UserId end, @Exception, @StackTrace); </commandText>
<parameter name="@TimeStamp" layout="${date}" />
<parameter name="@Level" layout="${level}" />
<parameter name="@Logger" layout="${logger}" />
<parameter name="@Message" layout="${message}" />
<parameter name="@UserId" layout="${mdc:user_id}" />
<parameter name="@Exception" layout="${exception}" />
<parameter name="@StackTrace" layout="${stacktrace}" />
<dbProvider>System.Data.SqlClient</dbProvider>
</target>
</targets>
<rules>
<!-- I am adding my 3 logging rules here -->
<logger name="*" minlevel="Debug" writeTo="database" />
<logger name="*" minlevel="Trace" writeTo="logfile" />
<logger name="*" minlevel="Trace" writeTo="eventlog" />
</rules>
</nlog>
We will targeting to log into three places.
database
txt file
Event Viewer
Your config look like below.
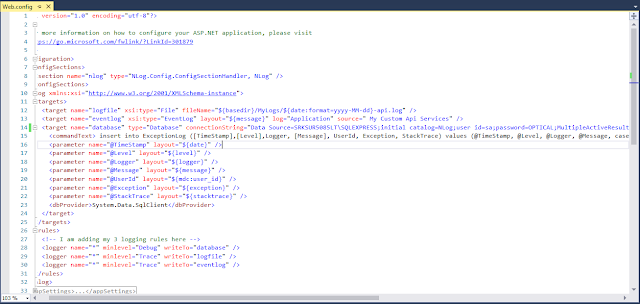
Step 6 : We need to create our DB Script as shown below
CREATE TABLE [dbo].[exceptionlog]
(
[id] [INT] IDENTITY(1, 1) NOT NULL,
[timestamp] [DATETIME] NOT NULL,
[level] [VARCHAR](100) NOT NULL,
[logger] [VARCHAR](1000) NOT NULL,
[message] [VARCHAR](3600) NOT NULL,
[userid] [INT] NULL,
[exception] [VARCHAR](3600) NULL,
[stacktrace] [VARCHAR](3600) NULL,
CONSTRAINT [PK_ExceptionLog] PRIMARY KEY CLUSTERED ( [id] ASC )WITH (
pad_index = OFF, statistics_norecompute = OFF, ignore_dup_key = OFF,
allow_row_locks = on, allow_page_locks = on) ON [PRIMARY]
)
ON [PRIMARY]
Step 7 : Now, go to your home controller and add following code in index method.
using NLog;
using System;
using System.Web.Mvc;
namespace NLogTestApp.Controllers
{
public class HomeController : Controller
{
private static Logger logger = LogManager.GetCurrentClassLogger();
public ActionResult Index()
{
ViewBag.Title = "Home Page";
logger.Info("Hello we have visited the Index view" + Environment.NewLine + DateTime.Now);
return View();
}
}
}
Now, run the application.
data-ad-format="auto" And open your SQL. You will see the following logs inserted in your database.
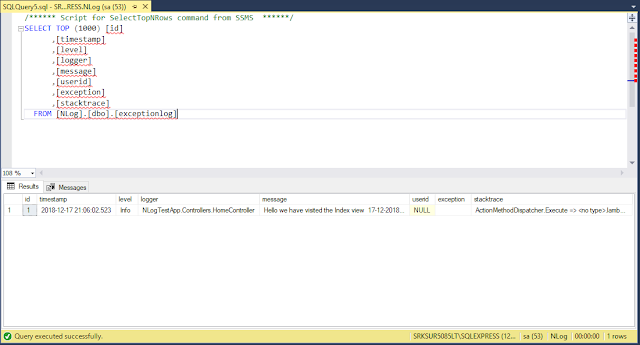
You can see this in your Event Viewer.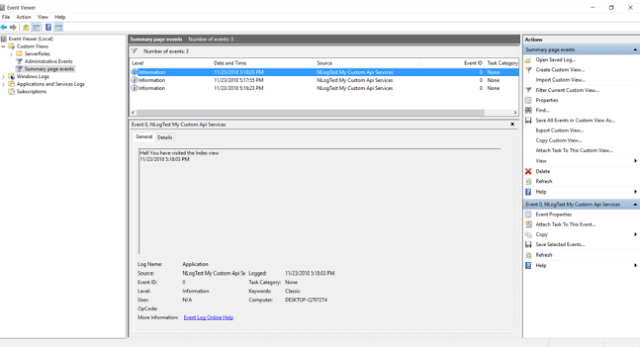
You can see the following in your text file.
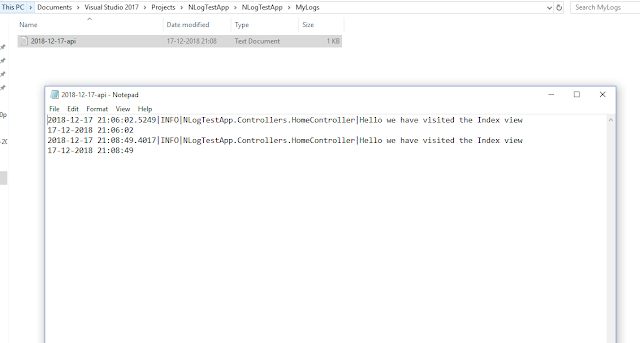
When you are working with WEPAPI that time NLog is very useful and easy to apply.
NLog is a flexible and free logging platform for various .NET platforms, including .NET standard. NLog is easy to apply and it includes several targets (database, file, event viewer).
Which platform support it?
.NET Framework 3.5, 4, 4.5, 4.6 & 4.7
.NET Framework 4 client profile
Xamarin Android
Xamarin iOS
Windows Phone 8
Silver light 4 and 5
Mono 4
ASP.NET 4 (NLog.Web package)
ASP.NET Core (NLog.Web.AspNetCore package)
.NET Core (NLog.Extensions.Logging package)
.NET Standard 1.x - NLog 4.5
.NET Standard 2.x - NLog 4.5
UWP - NLog 4.5
There are several log levels.
Fatal : Something terrible occurred; the application is going down
Error : Something fizzled; the application might possibly proceed
Warn : Something surprising; the application will proceed
Info : Normal conduct like mail sent, client refreshed profile and so on.
Debug : For troubleshooting; the executed question, the client confirmed, session terminated
Trace : For follow troubleshooting; start technique X, end strategy X
Where we can log?
Console
Database
Event Viewer Log
Files
Network
Implementation of NLog
Step 1 : Open Visual Studio. Select File > New > Project.
Step 2 : Select Web> ASP.NET Web Application > Give your project name "NLogTestApp", and then click OK.
Step 3 : Select Web API and click OK.
Step 4 : Now, we add NLog in our project. Right-click on your project solution, click on "Manage NugGet Packages". Click on the "Browse" link button and in the search box, type nlog. It will show you NLogDll as shown below. Just install this dll.
After that, when you click "Install", you will get the following window, i.e., Preview Changes.
In this popup window, click the OK button. After it is successfully installed, you will get the Nlog dll in your project reference.
Step 5 : Now, add the following code to your config file.
<configSections>
<section name="nlog" type="NLog.Config.ConfigSectionHandler, NLog" />
</configSections>
<nlog xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<targets>
<target name="logfile" xsi:type="File" fileName="${basedir}/MyLogs/${date:format=yyyy-MM-dd}-api.log" />
<target name="eventlog" xsi:type="EventLog" layout="${message}" log="Application" source=" My Custom Api Services" />
<target name="database" type="Database" connectionString="Data Source=SRKSUR5085LT\SQLEXPRESS;initial catalog=NLog;user id=sa;password=OPTICAL;MultipleActiveResultSets=True;">
<commandText> insert into ExceptionLog ([TimeStamp],[Level],Logger, [Message], UserId, Exception, StackTrace) values (@TimeStamp, @Level, @Logger, @Message, case when len(@UserID) = 0 then null else @UserId end, @Exception, @StackTrace); </commandText>
<parameter name="@TimeStamp" layout="${date}" />
<parameter name="@Level" layout="${level}" />
<parameter name="@Logger" layout="${logger}" />
<parameter name="@Message" layout="${message}" />
<parameter name="@UserId" layout="${mdc:user_id}" />
<parameter name="@Exception" layout="${exception}" />
<parameter name="@StackTrace" layout="${stacktrace}" />
<dbProvider>System.Data.SqlClient</dbProvider>
</target>
</targets>
<rules>
<!-- I am adding my 3 logging rules here -->
<logger name="*" minlevel="Debug" writeTo="database" />
<logger name="*" minlevel="Trace" writeTo="logfile" />
<logger name="*" minlevel="Trace" writeTo="eventlog" />
</rules>
</nlog>
We will targeting to log into three places.
database
txt file
Event Viewer
Your config look like below.
Step 6 : We need to create our DB Script as shown below
CREATE TABLE [dbo].[exceptionlog]
(
[id] [INT] IDENTITY(1, 1) NOT NULL,
[timestamp] [DATETIME] NOT NULL,
[level] [VARCHAR](100) NOT NULL,
[logger] [VARCHAR](1000) NOT NULL,
[message] [VARCHAR](3600) NOT NULL,
[userid] [INT] NULL,
[exception] [VARCHAR](3600) NULL,
[stacktrace] [VARCHAR](3600) NULL,
CONSTRAINT [PK_ExceptionLog] PRIMARY KEY CLUSTERED ( [id] ASC )WITH (
pad_index = OFF, statistics_norecompute = OFF, ignore_dup_key = OFF,
allow_row_locks = on, allow_page_locks = on) ON [PRIMARY]
)
ON [PRIMARY]
Step 7 : Now, go to your home controller and add following code in index method.
using NLog;
using System;
using System.Web.Mvc;
namespace NLogTestApp.Controllers
{
public class HomeController : Controller
{
private static Logger logger = LogManager.GetCurrentClassLogger();
public ActionResult Index()
{
ViewBag.Title = "Home Page";
logger.Info("Hello we have visited the Index view" + Environment.NewLine + DateTime.Now);
return View();
}
}
}
Now, run the application.
data-ad-format="auto" And open your SQL. You will see the following logs inserted in your database.
You can see this in your Event Viewer.
You can see the following in your text file.
When you are working with WEPAPI that time NLog is very useful and easy to apply.